41paramValues
<h1>el paramValues 기본객체</h1>
<p>0 : <%= request.getParameterValues("param1")[0] %></p>
<p>1 : <%= request.getParameterValues("param1")[1] %></p>
<p>2 : <%= request.getParameterValues("param1")[2] %></p>
<hr>
<p>\${paramValues.param1[0] } : ${paramValues.param1[0] }</p>
<p>\${paramValues.param1[1] } : ${paramValues.param1[1] }</p>
<p>\${paramValues.param1[2] } : ${paramValues.param1[2] }</p>
< el paramValues 기본객체 >
el 로 안하면 <%= request.getParameterValues("param1")[0] %> 로 꺼내야하고,
el 로 하면 ${paramValues.param1[0]} 로 반환하면 된다는 거지
- paramValues : 요청 파라미터의 <파라미터 이름, 값 배열> 매핑을 저장한 Map 객체이다. 파라미터 값 타입은 String[] 로서 request.getParamaterValues(name) 과 동일
같은 이름으로 여러개의 값을 넣으면 배열로 저장된다
42from, view
from
<form action="42view.jsp" method="post">
<input type="text" name="name" id="" placeholder="이름"> <br>
<input type="checkbox" name="food" value="pizza" id="">pizza
<br>
<input type="checkbox" name="food" value="cake" id="">cake
<br>
<input type="checkbox" name="food" value="milk" id="">milk
<br>
<input type="submit" value="전송">
</form>
그냥 체크박스 선택창, action 으로 view 로 전달
view
<p>name : ${param.name }</p>
<p>food 1 : ${paramValues.food[0] }</p>
<p>food 2 : ${paramValues.food[1] }</p>
<p>food 3 : ${paramValues.food[2] }</p>
<hr>
<c:forEach items="${paramValues.food }" var="food" varStatus="status">
<p>food ${status.count } : ${food }</p>
</c:forEach>
반환
${paramValues.name[index] }
아래는 jstl 인데 이때 <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> import 해야함
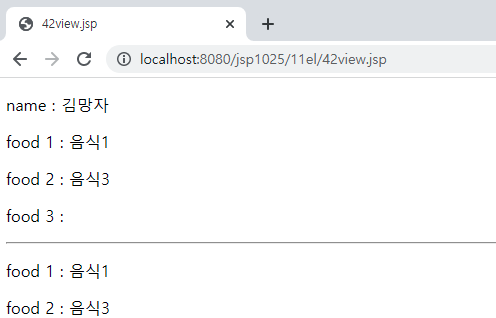
43header
<el header 기본객체>
<p>${header.connection }</p>
<p>${header.host }</p>
<p>${header["user-agent"] }</p>
<p>${header["accept"] }</p>
<헤더이름, 값> 매핑을 저장한 map
request.getHeader(name) 과 결과 동일

44cookie
< el cookie 기본객체 >
<p>${cookie.JSESSIONID }</p>
<p>${cookie.JSESSIONID.name }</p>
<p>${cookie.JSESSIONID.value }</p>
<쿠키이름, cookie> 매핑을 저장한 map 객체
request.getHeaders(name) 과 결과 동일

45pageContext
<el pageContext 기본객체>
<p>${pageContext }</p>
<p>${pageContext.request }</p>
<p>${pageContext.session }</p>
<p>${pageContext.request.cookies[0].name }</p>
<p>${pageContext.request.cookies[0].value }</p>
<p><%= request.getContextPath() %></p>
<p>${pageContext.request.contextPath }</p>
map 이 아닌 그냥 객체
pageContext 로부터 찾기위해
jsp 의 다른 기본객체를 자바코드가 아닌 el 을 통해서 얻기 위해서 pageContext 를 찾아서
<%= request.getContextPath() %>
${pageContext.request.contextPath }
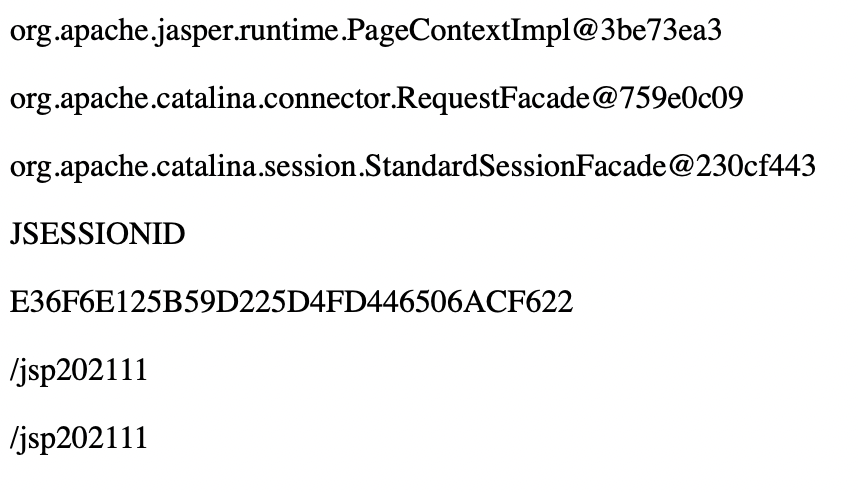
46arithmetic-operator
<el 산술연산>
<%
pageContext.setAttribute("num1", 5);
pageContext.setAttribute("num2", 3);
%>
<p>num1 : ${num1 }</p>
<p>num2 : ${num2 }</p>
<p>num1 + num2 : ${num1 + num2 }</p>
<p>num1 - num2 : ${num1 - num2 }</p>
<p>num1 * num2 : ${num1 * num2 }</p>
<p>num1 / num2 : ${num1 / num2 }</p>
<p>num1 / num2 : ${num1 div num2 }</p>
<p>num1 % num2 : ${num1 % num2 }</p>
<p>num1 % num2 : ${num1 mod num2 }</p>
<hr>
<%
pageContext.setAttribute("num3", "5");
pageContext.setAttribute("num4", "3");
%>
<p>num3 + num4 : ${num3 + num4 }</p>
<p>num3 num4 연결 : ${num3 }${num4 }</p>
<hr>
<%
pageContext.setAttribute("num5", "five");
pageContext.setAttribute("num6", "three");
%>
<p>num5 num6 연결 : ${num5 }${num6 }</p>
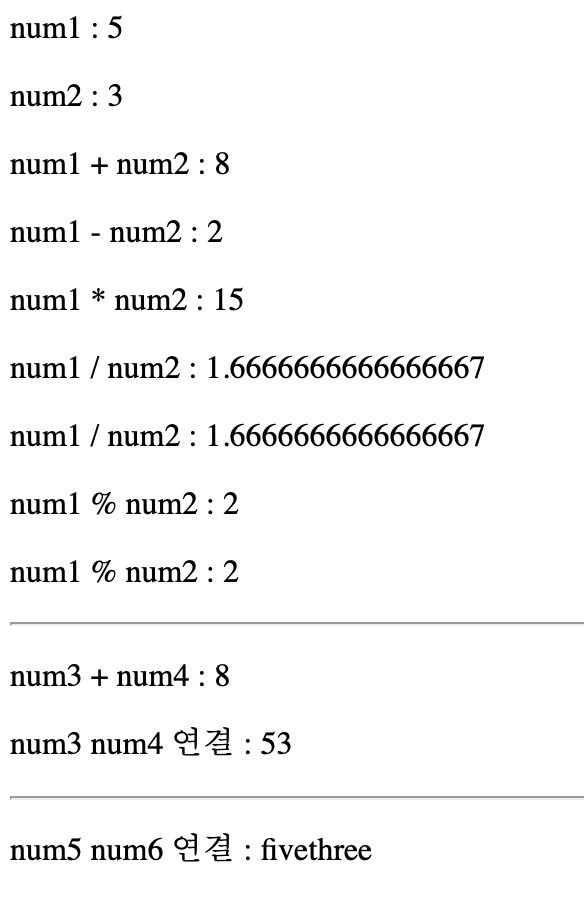
- setAttribute 으로 num1 에 5를 저장, num2 에 3를 저장
연산하면 된다 이거 저번에 형변환 해야된다 거기서 배웠었음
/, div - 나누기에서 소수점까지
%, mod - 나누기에서 나머지
- setAttribute 으로 num3 에 5를 저장, num4 에 3를 저장
더하기와 그냥 이어서 쓰기 차이
- num5 에 five 를 저장, num6 에 three 를 저장
이건 문자열, 그냥 이어서 쓰면 이어서 써짐
47arithmetic-ex
<p>a + b: ${param.a + param.b } </p>
<p>a - b: ${param.a - param.b }</p>
<p>a * b: ${param.a * param.b } </p>
<p>a / b: ${param.a / param.b } </p>
<p>a % b: ${param.a % param.b }</p>
param.a , param.b 로 연산도 가능
48compare
<%
pageContext.setAttribute("n1", 5);
pageContext.setAttribute("n2", 3);
%>
<p>n1 == n2 : ${n1 == n2 }</p>
<p>n1 == n2 : ${n1 eq n2 }</p>
<p>n1 != n2 : ${n1 != n2 }</p>
<p>n1 != n2 : ${n1 ne n2 }</p>
<p>n1 > n2 : ${n1 > n2 }</p>
<p>n1 > n2 : ${n1 gt n2 }</p>
<p>n1 >= n2 : ${n1 >= n2 }</p>
<p>n1 >= n2 : ${n1 ge n2 }</p>
<p>n1 < n2 : ${n1 < n2 }</p>
<p>n1 < n2 : ${n1 lt n2 }</p>
<p>n1 <= n2 : ${n1 <= n2 }</p>
<p>n1 <= n2 : ${n1 le n2 }</p>
동등비교, 대소비교 - 결과가 boolean형 (true, false) 로 나옴
n1에 5를, n2에 3을 저장
<동등비교>
==, eq
!=, ne
<대소비교>
>, gt
>=, ge
<, lt
<=, le
49compare-ex
<p>n1 == n2 : ${param.n1 == param.n2 } </p>
<p>n1 != n2 : ${param.n1 != param.n2 }</p>
<p>n1 > n2 : ${param.n1 gt param.n2 }</p>
<p>n1 >= n2 : ${param.n1 ge param.n2 }</p>
<p>n1 < n2 : ${param.n1 lt param.n2 }</p>
<p>n1 <= n2 : ${param.n1 le param.n2 }</p>
param. 달고 해보기
50logical-operator
<h1>논리 연산자 </h1>
<h2>and : 두 항이 true일 때만 true, 나머지 모두 false</h2>
<p>\${true && true } : ${true && true }</p>
<p>\${true && false } : ${true && false }</p>
<p>\${false && true } : ${false && true }</p>
<p>\${false && false } : ${false && false }</p>
<h2>or : 두 항이 false일 때만 false, 나머지 모두 true</h2>
<p>\${true || true } : ${true || true }</p>
<p>\${true || false } : ${true || false }</p>
<p>\${false || true } : ${false || true }</p>
<p>\${false || false } : ${false || false }</p>
<h2>not : false --> true, true --> false</h2>
<p>\${! false } : ${! false }</p>
<p>\${! true } : ${! true }</p>
<hr>
<h2>and</h2>
<p>\${true and true } : ${true and true }</p>
<p>\${true and false } : ${true and false }</p>
<p>\${false and true } : ${false and true }</p>
<p>\${false and false } : ${false and false }</p>
<h2>or</h2>
<p>\${true or true } : ${true or true }</p>
<p>\${true or false } : ${true or false }</p>
<p>\${false or true } : ${false or true }</p>
<p>\${false or false } : ${false or false }</p>
<h2>not</h2>
<p>\${not false } : ${not false }</p>
<p>\${not true } : ${not true }</p>
논리연산자
and(&&, and): 두 항이 true 일때만 true, 나머지 false
or(||, or): 두 항이 false 일때만 false, 나머지 true
not(!, not): false 는 true 로, true 는 false 로 바꾸기
51logical-ex
<%
pageContext.setAttribute("x", 6);
pageContext.setAttribute("y", 3);
%>
<p>${x lt 10 and y gt 1 }</p>
<p>${x < 10 && y > 1 }</p>
<p>${x eq 5 or y eq 5 }</p>
<p>${x == 5 || y == 5 }</p>
<p>${not (x eq y) }</p>
<p>${! (x == y) }</p>
그냥 예제
52empty
<h1>empty</h1>
<ul>
<li>null이면 true</li>
<li>빈 string("")이면 true</li>
<li>길이가 0인 배열이면 true</li>
<li>size가 0인 map은 true</li>
<li>size가 0인 (list, set)이면 true</li>
<li>나머지 false</li>
</ul>
<hr>
<%
pageContext.setAttribute("str", "");
%>
<p>${empty str }</p>
<%
pageContext.setAttribute("arr", new String[] {});
%>
<p>${empty arr }</p>
<%
pageContext.setAttribute("map", new HashMap());
%>
<p>${empty map }</p>
<%
pageContext.setAttribute("list", new ArrayList());
%>
<p>${empty list }</p>
<%
pageContext.setAttribute("set", new HashSet());
%>
<p>${empty set }</p>
<%
pageContext.setAttribute("var1", "val1");
%>
<p>${empty var2 }</p>
<hr>
<h1>값이 있는 지 확인하고 싶을 때는 not empty (! empty)</h1>
<empty>
null ( 빈 string("") , 길이가 0인 배열 , size가 0인 map , size가 0인 (list, set) ) 이면 true
나머지 false
값이 있는지 확인하고 싶을 때 사용
53ternary
<h1>삼항 연산자 </h1>
<%
pageContext.setAttribute("age", 18);
%>
<p>${(age > 18) ? "old enough" : "too young" }</p>
삼항연산자
age 에 18 저장, age 가 18 초과이면 old enough, 이하이면 too young 반환
54js
<h1>javascript</h1>
<script>
console.log("hello js");
let name = "trump";
let age = 22;
let desc = `i'm \${name} and i'm \${age}`;
console.log(desc);
</script>
script
attribute 값이 bean, 객체, map, list 일때 점연산자, 네모괄호 연산자로 (자바코드없이) 11개 기본객체를 attribute 받을 때는 기본객체로부터 받는다
11개 기본객체를 attribute 받을 때는 기본객체부터 받음
자바코드랑 사용할 때 유의할 점
template literal - 작은따옴표(')나 큰따옴표(") 대신 백틱(`)(grave accent) 로 감싸줌
백틱(`) 안에 el 표현이 있는데, 이를 jsp 에 넣으려면 백슬래시(\) 를 앞에 써야 함
<script> 안에서 el 을 쓰려면 앞에 백슬래시(\) 를 붙여서 써야 함 (jQuery 도 마찬가지)
보통은 jsp 와 js 를 분리해서 작성한다
* 백슬래시(\) 는 escape 문자로 표현식 앞에 백슬래시(\) 를 붙이면 해당 표현식은 파싱되지 않고 문자열 그대로 나온다
** 맥북에서 백틱 쓰는 법 - 영문일때는 그냥 입력해도 됨, 한글일때는 option과 함께 입력
55js
<h1>javascript 연습</h1>
<%
pageContext.setAttribute("name", "korea");
pageContext.setAttribute("city", "seoul");
%>
<script>
let name = "france";
let city = "paris";
let info1 = `name : ${name}, city: ${city}`; // 코드 작성
let info2 = `name : \${name}, city: \${city}`; // 코드 작성
console.log(info1); // name : korea, city : seoul
console.log(info2); // name : france, city : paris
</script>
info 1 html 소스코드 자체가 (톰캣) info2 자바스크립트 변수에 들어있음
자바스크립트 변수를 쓰고싶어서 내가 쓰고싶은게 ${name}, ${city} 그래서 백슬래시 붙임
56string
<h1>string</h1>
<p>${param.name == "donald" }</p>
<p>${param.name == 'donald' }</p>
el 은 자바코드가 아니라서 - 자바코드는 string 값을 "" 로 표현
el 에서는 큰따옴표, 작은따옴표 둘다 가능함
'course 2021 > JSP' 카테고리의 다른 글
JSP16 - 13customTag (0) | 2021.11.24 |
---|---|
JSP15 - 12jstl (0) | 2021.11.22 |
JSP13 - 11el(2) (0) | 2021.11.20 |
JSP12 - 11el (0) | 2021.11.19 |
JSP11 - 10scope (0) | 2021.11.19 |
41paramValues
<h1>el paramValues 기본객체</h1>
<p>0 : <%= request.getParameterValues("param1")[0] %></p>
<p>1 : <%= request.getParameterValues("param1")[1] %></p>
<p>2 : <%= request.getParameterValues("param1")[2] %></p>
<hr>
<p>\${paramValues.param1[0] } : ${paramValues.param1[0] }</p>
<p>\${paramValues.param1[1] } : ${paramValues.param1[1] }</p>
<p>\${paramValues.param1[2] } : ${paramValues.param1[2] }</p>
< el paramValues 기본객체 >
el 로 안하면 <%= request.getParameterValues("param1")[0] %> 로 꺼내야하고,
el 로 하면 ${paramValues.param1[0]} 로 반환하면 된다는 거지
- paramValues : 요청 파라미터의 <파라미터 이름, 값 배열> 매핑을 저장한 Map 객체이다. 파라미터 값 타입은 String[] 로서 request.getParamaterValues(name) 과 동일
같은 이름으로 여러개의 값을 넣으면 배열로 저장된다
42from, view
from
<form action="42view.jsp" method="post">
<input type="text" name="name" id="" placeholder="이름"> <br>
<input type="checkbox" name="food" value="pizza" id="">pizza
<br>
<input type="checkbox" name="food" value="cake" id="">cake
<br>
<input type="checkbox" name="food" value="milk" id="">milk
<br>
<input type="submit" value="전송">
</form>
그냥 체크박스 선택창, action 으로 view 로 전달
view
<p>name : ${param.name }</p>
<p>food 1 : ${paramValues.food[0] }</p>
<p>food 2 : ${paramValues.food[1] }</p>
<p>food 3 : ${paramValues.food[2] }</p>
<hr>
<c:forEach items="${paramValues.food }" var="food" varStatus="status">
<p>food ${status.count } : ${food }</p>
</c:forEach>
반환
${paramValues.name[index] }
아래는 jstl 인데 이때 <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> import 해야함
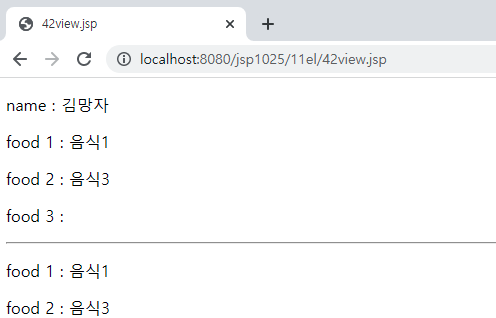
43header
<el header 기본객체>
<p>${header.connection }</p>
<p>${header.host }</p>
<p>${header["user-agent"] }</p>
<p>${header["accept"] }</p>
<헤더이름, 값> 매핑을 저장한 map
request.getHeader(name) 과 결과 동일

44cookie
< el cookie 기본객체 >
<p>${cookie.JSESSIONID }</p>
<p>${cookie.JSESSIONID.name }</p>
<p>${cookie.JSESSIONID.value }</p>
<쿠키이름, cookie> 매핑을 저장한 map 객체
request.getHeaders(name) 과 결과 동일

45pageContext
<el pageContext 기본객체>
<p>${pageContext }</p>
<p>${pageContext.request }</p>
<p>${pageContext.session }</p>
<p>${pageContext.request.cookies[0].name }</p>
<p>${pageContext.request.cookies[0].value }</p>
<p><%= request.getContextPath() %></p>
<p>${pageContext.request.contextPath }</p>
map 이 아닌 그냥 객체
pageContext 로부터 찾기위해
jsp 의 다른 기본객체를 자바코드가 아닌 el 을 통해서 얻기 위해서 pageContext 를 찾아서
<%= request.getContextPath() %>
${pageContext.request.contextPath }
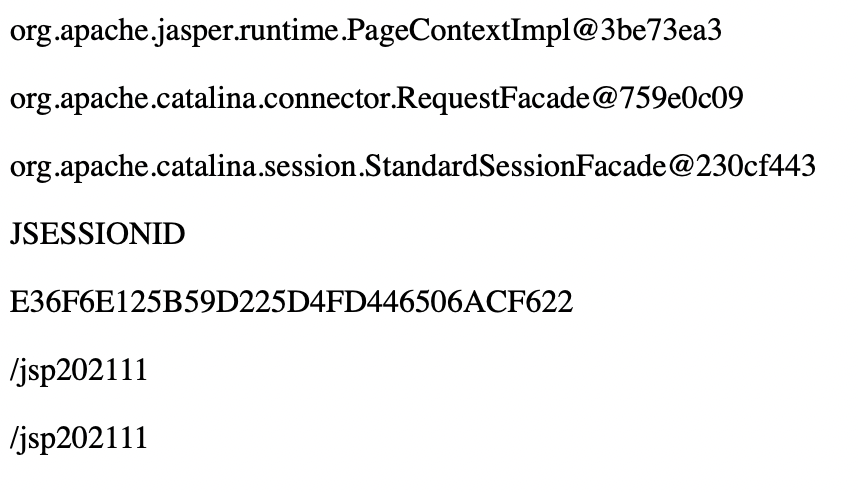
46arithmetic-operator
<el 산술연산>
<%
pageContext.setAttribute("num1", 5);
pageContext.setAttribute("num2", 3);
%>
<p>num1 : ${num1 }</p>
<p>num2 : ${num2 }</p>
<p>num1 + num2 : ${num1 + num2 }</p>
<p>num1 - num2 : ${num1 - num2 }</p>
<p>num1 * num2 : ${num1 * num2 }</p>
<p>num1 / num2 : ${num1 / num2 }</p>
<p>num1 / num2 : ${num1 div num2 }</p>
<p>num1 % num2 : ${num1 % num2 }</p>
<p>num1 % num2 : ${num1 mod num2 }</p>
<hr>
<%
pageContext.setAttribute("num3", "5");
pageContext.setAttribute("num4", "3");
%>
<p>num3 + num4 : ${num3 + num4 }</p>
<p>num3 num4 연결 : ${num3 }${num4 }</p>
<hr>
<%
pageContext.setAttribute("num5", "five");
pageContext.setAttribute("num6", "three");
%>
<p>num5 num6 연결 : ${num5 }${num6 }</p>
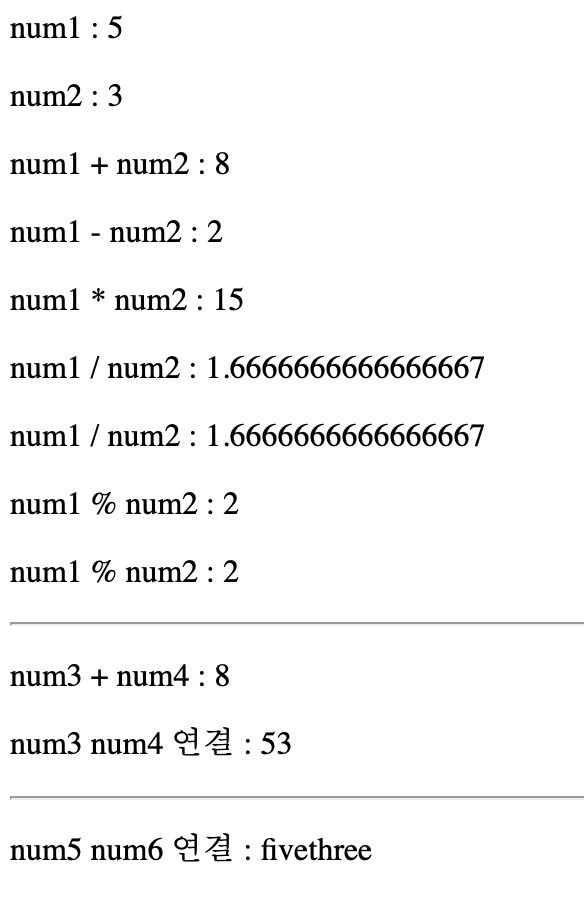
- setAttribute 으로 num1 에 5를 저장, num2 에 3를 저장
연산하면 된다 이거 저번에 형변환 해야된다 거기서 배웠었음
/, div - 나누기에서 소수점까지
%, mod - 나누기에서 나머지
- setAttribute 으로 num3 에 5를 저장, num4 에 3를 저장
더하기와 그냥 이어서 쓰기 차이
- num5 에 five 를 저장, num6 에 three 를 저장
이건 문자열, 그냥 이어서 쓰면 이어서 써짐
47arithmetic-ex
<p>a + b: ${param.a + param.b } </p>
<p>a - b: ${param.a - param.b }</p>
<p>a * b: ${param.a * param.b } </p>
<p>a / b: ${param.a / param.b } </p>
<p>a % b: ${param.a % param.b }</p>
param.a , param.b 로 연산도 가능
48compare
<%
pageContext.setAttribute("n1", 5);
pageContext.setAttribute("n2", 3);
%>
<p>n1 == n2 : ${n1 == n2 }</p>
<p>n1 == n2 : ${n1 eq n2 }</p>
<p>n1 != n2 : ${n1 != n2 }</p>
<p>n1 != n2 : ${n1 ne n2 }</p>
<p>n1 > n2 : ${n1 > n2 }</p>
<p>n1 > n2 : ${n1 gt n2 }</p>
<p>n1 >= n2 : ${n1 >= n2 }</p>
<p>n1 >= n2 : ${n1 ge n2 }</p>
<p>n1 < n2 : ${n1 < n2 }</p>
<p>n1 < n2 : ${n1 lt n2 }</p>
<p>n1 <= n2 : ${n1 <= n2 }</p>
<p>n1 <= n2 : ${n1 le n2 }</p>
동등비교, 대소비교 - 결과가 boolean형 (true, false) 로 나옴
n1에 5를, n2에 3을 저장
<동등비교>
==, eq
!=, ne
<대소비교>
>, gt
>=, ge
<, lt
<=, le
49compare-ex
<p>n1 == n2 : ${param.n1 == param.n2 } </p>
<p>n1 != n2 : ${param.n1 != param.n2 }</p>
<p>n1 > n2 : ${param.n1 gt param.n2 }</p>
<p>n1 >= n2 : ${param.n1 ge param.n2 }</p>
<p>n1 < n2 : ${param.n1 lt param.n2 }</p>
<p>n1 <= n2 : ${param.n1 le param.n2 }</p>
param. 달고 해보기
50logical-operator
<h1>논리 연산자 </h1>
<h2>and : 두 항이 true일 때만 true, 나머지 모두 false</h2>
<p>\${true && true } : ${true && true }</p>
<p>\${true && false } : ${true && false }</p>
<p>\${false && true } : ${false && true }</p>
<p>\${false && false } : ${false && false }</p>
<h2>or : 두 항이 false일 때만 false, 나머지 모두 true</h2>
<p>\${true || true } : ${true || true }</p>
<p>\${true || false } : ${true || false }</p>
<p>\${false || true } : ${false || true }</p>
<p>\${false || false } : ${false || false }</p>
<h2>not : false --> true, true --> false</h2>
<p>\${! false } : ${! false }</p>
<p>\${! true } : ${! true }</p>
<hr>
<h2>and</h2>
<p>\${true and true } : ${true and true }</p>
<p>\${true and false } : ${true and false }</p>
<p>\${false and true } : ${false and true }</p>
<p>\${false and false } : ${false and false }</p>
<h2>or</h2>
<p>\${true or true } : ${true or true }</p>
<p>\${true or false } : ${true or false }</p>
<p>\${false or true } : ${false or true }</p>
<p>\${false or false } : ${false or false }</p>
<h2>not</h2>
<p>\${not false } : ${not false }</p>
<p>\${not true } : ${not true }</p>
논리연산자
and(&&, and): 두 항이 true 일때만 true, 나머지 false
or(||, or): 두 항이 false 일때만 false, 나머지 true
not(!, not): false 는 true 로, true 는 false 로 바꾸기
51logical-ex
<%
pageContext.setAttribute("x", 6);
pageContext.setAttribute("y", 3);
%>
<p>${x lt 10 and y gt 1 }</p>
<p>${x < 10 && y > 1 }</p>
<p>${x eq 5 or y eq 5 }</p>
<p>${x == 5 || y == 5 }</p>
<p>${not (x eq y) }</p>
<p>${! (x == y) }</p>
그냥 예제
52empty
<h1>empty</h1>
<ul>
<li>null이면 true</li>
<li>빈 string("")이면 true</li>
<li>길이가 0인 배열이면 true</li>
<li>size가 0인 map은 true</li>
<li>size가 0인 (list, set)이면 true</li>
<li>나머지 false</li>
</ul>
<hr>
<%
pageContext.setAttribute("str", "");
%>
<p>${empty str }</p>
<%
pageContext.setAttribute("arr", new String[] {});
%>
<p>${empty arr }</p>
<%
pageContext.setAttribute("map", new HashMap());
%>
<p>${empty map }</p>
<%
pageContext.setAttribute("list", new ArrayList());
%>
<p>${empty list }</p>
<%
pageContext.setAttribute("set", new HashSet());
%>
<p>${empty set }</p>
<%
pageContext.setAttribute("var1", "val1");
%>
<p>${empty var2 }</p>
<hr>
<h1>값이 있는 지 확인하고 싶을 때는 not empty (! empty)</h1>
<empty>
null ( 빈 string("") , 길이가 0인 배열 , size가 0인 map , size가 0인 (list, set) ) 이면 true
나머지 false
값이 있는지 확인하고 싶을 때 사용
53ternary
<h1>삼항 연산자 </h1>
<%
pageContext.setAttribute("age", 18);
%>
<p>${(age > 18) ? "old enough" : "too young" }</p>
삼항연산자
age 에 18 저장, age 가 18 초과이면 old enough, 이하이면 too young 반환
54js
<h1>javascript</h1>
<script>
console.log("hello js");
let name = "trump";
let age = 22;
let desc = `i'm \${name} and i'm \${age}`;
console.log(desc);
</script>
script
attribute 값이 bean, 객체, map, list 일때 점연산자, 네모괄호 연산자로 (자바코드없이) 11개 기본객체를 attribute 받을 때는 기본객체로부터 받는다
11개 기본객체를 attribute 받을 때는 기본객체부터 받음
자바코드랑 사용할 때 유의할 점
template literal - 작은따옴표(')나 큰따옴표(") 대신 백틱(`)(grave accent) 로 감싸줌
백틱(`) 안에 el 표현이 있는데, 이를 jsp 에 넣으려면 백슬래시(\) 를 앞에 써야 함
<script> 안에서 el 을 쓰려면 앞에 백슬래시(\) 를 붙여서 써야 함 (jQuery 도 마찬가지)
보통은 jsp 와 js 를 분리해서 작성한다
* 백슬래시(\) 는 escape 문자로 표현식 앞에 백슬래시(\) 를 붙이면 해당 표현식은 파싱되지 않고 문자열 그대로 나온다
** 맥북에서 백틱 쓰는 법 - 영문일때는 그냥 입력해도 됨, 한글일때는 option과 함께 입력
55js
<h1>javascript 연습</h1>
<%
pageContext.setAttribute("name", "korea");
pageContext.setAttribute("city", "seoul");
%>
<script>
let name = "france";
let city = "paris";
let info1 = `name : ${name}, city: ${city}`; // 코드 작성
let info2 = `name : \${name}, city: \${city}`; // 코드 작성
console.log(info1); // name : korea, city : seoul
console.log(info2); // name : france, city : paris
</script>
info 1 html 소스코드 자체가 (톰캣) info2 자바스크립트 변수에 들어있음
자바스크립트 변수를 쓰고싶어서 내가 쓰고싶은게 ${name}, ${city} 그래서 백슬래시 붙임
56string
<h1>string</h1>
<p>${param.name == "donald" }</p>
<p>${param.name == 'donald' }</p>
el 은 자바코드가 아니라서 - 자바코드는 string 값을 "" 로 표현
el 에서는 큰따옴표, 작은따옴표 둘다 가능함
'course 2021 > JSP' 카테고리의 다른 글
JSP16 - 13customTag (0) | 2021.11.24 |
---|---|
JSP15 - 12jstl (0) | 2021.11.22 |
JSP13 - 11el(2) (0) | 2021.11.20 |
JSP12 - 11el (0) | 2021.11.19 |
JSP11 - 10scope (0) | 2021.11.19 |